This guide will teach you to build Java application using the maven build tool. This is a step-by-step guide for beginners where we will discuss all the important aspects of a java application build from a DevOps perspective.
As a devops engineer, learning the application build process is very important as you will be actively working on CI/CD tasks. If you have good knowledge of java applications and the build process, you can collaborate easily with developers and make everyone's life easy in terms of setting up CI/CD for java based applications.
What is Maven?
Maven is one of the open-source Java build tools developed by Apache Software Foundation. It can compile, test, and package a java program into .jar
or .war
format.
Maven makes use of the pom.xml file to build java projects.
Project Object Model (POM) is an XML file that contains the java project details, configurations, and settings required for maven to build the project.
The pom.xml
file is present in the root of the java project directory. Primarily it contains the project dependencies.
For example, when a developer wants to implement a PostgreSQL database connectivity functionality, he will make use of the PostgreSQL JDBC Driver dependency from the maven repository by adding it to the pom.xml file.
So when you build the code with maven, it reads the pom.xml
file and downloads all the dependencies from the maven repository. Dependencies could be third-party libraries from the public Maven Repository or common libraries hosted within an organization's private maven repository. You can compare it with Python pip, Nodejs npm, or Ruby gems
Commonly organizations use Sonatyope nexus as a private hosted maven repository.
By default, maven uses the public repository but if you have in-house private maven repositories, you configure custom maven repository URLs in settings.xml
maven configuration present in the maven installation directory. for example, /opt/apache-maven-3.8.6/conf/settings.xml
Maven Prerequisites
For maven to work you need the following installed on your system
- Java JDK
- Maven
To install and configure JDK and maven, follow the maven installation guide.
Build Java Application Using Maven
For this example, we will be using the open-source java spring boot application named pet-clinic.
First, clone the application to your development machine or server.
git clone https://github.com/spring-projects/spring-petclinic.git
The code base has the following important folders and files. It is common in real-time project code as well.
- /src folder: This folder contains the source code based on the java spring framework.
- /src/tests folder: This folder contains the unit tests & integration tests of the code under the
tests
folder. - pom.xml file: It contains all the dependencies required for the pet-clinic applications. As it is an open-source application, all the dependencies are from the public maven repository.
To build the project, cd into the project root directory. In my case its spring-petclinic
. It should contain the pom.xml
file
cd spring-petclinic
From a CI perspective, we just have to build, test, and package the project to create a deployable artifact(jar file)
So commonly in the CI process, we build and package the java projects using the following maven command. It compiles the code, tests it, package it as a jar file in the target folder, and will also install(copy) the jar package in the local .m2
repository.
mvn clean install
After executing the above command, you will see a folder named target
in the root directory. Inside the target directory, you will see the packaged jar file as shown below. We call it a deployable artifact.
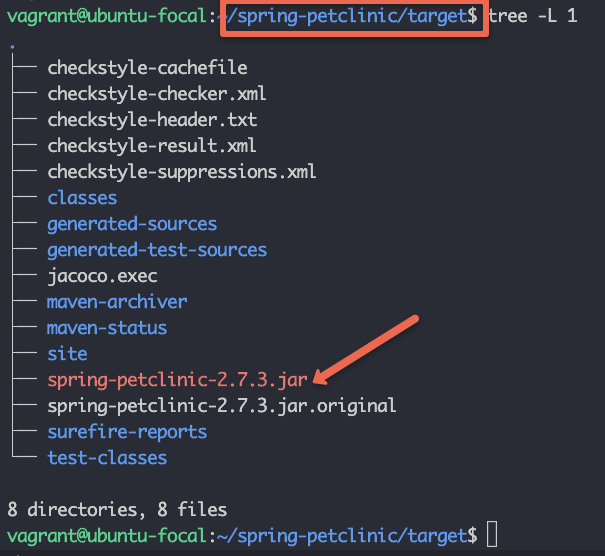
Even time you run mvn clean install
, it deletes target directory and packages from the local .m2
repository and replaces it with the latest build files and packages.
If you want to skip the test during build, you can add the -Dmaven.test.skip=true
parameter as shown below.
mvn clean install -Dmaven.test.skip=true
Now that you have understood how to build a java project using maven, let's look into the maven lifecycle. Few commands we don't have to use in the CI pipelines. However, it is good to know about the maven lifecycle commands and you can use them depending on your CI pipeline requirement.
Maven Lifecycle Explained
Let's take a look at each maven lifecycle phase in order. Each phase executes all the phases before it. For example, if you execute the third phase, one, two, and three get executed.
1. Maven Validate (mvn validate)
mvn validate
validates the maven project. It downloads all the required dependencies to the local .m2
repository.
2. Maven Compile (mvn compile)
mvn compile
compiles the java project. It runs validate first and then compiles the code.
3. Maven Test (mvn test)
mvn test
command runs the unit test that is part of the code. You can test classes individually, methods individually, or add patterns to run tests on all methods that match the pattern.
4. Maven Package (mvn package)
mvn package
commands compile the code, test it and finally package it in the required format (jar or war)
5. Maven Verify (mvn verify)
mvn verify command runs all the phases explained before in order and runs checks on integration tests and checkstyles if they are defined in the project.
6. Maven Install (mvn install)
mvn install
command installs the packaged code in the local maven repository.
7. Maven Deploy (mvn deploy)
mvn deploy
command, deploys the package to the remote maven repository. When you run deploy, it first runs validate, compile, test, package, verify, install, and then finally deploys the package to the remote maven repository.
Possible Maven Build Errors
java.lang.IllegalStateException: Unable to load cache item
If maven doesn't support the Java version, you will get the above error.
To rectify it, install the latest maven version that supports the installed Java version.
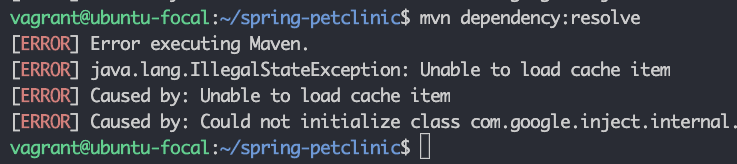
If you try to execute the maven command from the location where there is no pom.xml
file, you will get the following error.
The goal you specified requires a project to execute but there is no POM in this directory
To rectify this, execute the maven command from the folder that has the pom.xml file.
Maven Build FAQs
The following are the frequently asked questions about Maven Build.
Does mvn package run tests?
Yes. By default, the mvn package
command runs the test. However, you can add the flag -Dmaven.test.skip
to skip the tests.
What does Maven test do?
mvn test
runs all the unit tests for the java project.
Conclusion
As a Devops engineer, it is very important to understand the java build process if you are working on deploying java projects.
For most java projects, maven is used as a build tool for development and Continuous integration. So ensure, you have the same version of maven installed from the development to the CI environment.